Model View Controller architecture (or pattern) allows us to separate different parts of our applications into tiers to fulfill this need.
MVC Overview
Model View Controller architecture aims to separate an application into three parts:
Model: It is the business logic of an application. From an object oriented perspective it would consist of a set of classes that implement the critical functionality of an application from a business point of view.
View: It can consist of every type of interface given to the user. In ASP.NET the view is the set of web pages presented by a web application.
Controller: This part of the architecture is the most difficult to explain, hence the most difficult to implement in many platforms. The controller is the object that allows the manipulation of the view. Usually many applications implement Model-Controller tiers that contain the business logic along with the necessary code to manipulate a user interface. In an ASP.NET application the controller is implicitly represented by the code-behind or the server side code that generates the HTML presented to the user.
Implementing MVC in ASP.NET
A basic diagram that would help us understand perfectly the specific parts that implement the Model View Controller architecture in an ASP.NET application is presented below:
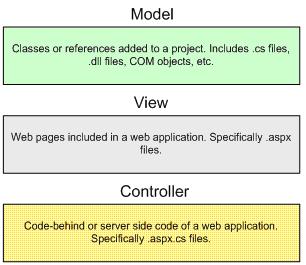
MVC Model Implementation
When implementing the business logic of an application it is a must to use a Class Library project in order to generate a .dll file that will encapsulate all the functionality. This is critical as we as professional developers would not like to jeopardize the source code of a software product by placing the actual .cs files as a reference in a web application.
This type of project can be easily created in Visual Studio 2005 under the Visual C# or Visual Basic tabs:
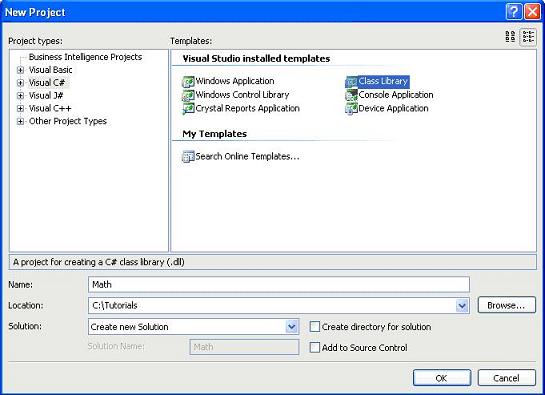
As a tutorial example we will develop a simple calculator under a new namespace we will call "Math".
Once the project is created we will add a class called Calculator:
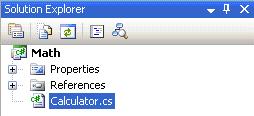
As the code is very simple and a sample is provided in this tutorial we will not get into much detail as far as how it is developed. The only important thing we need to mention is the way errors have to be handled in this class. Take a look at the following code:
1. protected float Divide(float fNumber1, float fNumber2)
2. {
3. if (fNumber2 == 0)
4. {
5. throw new Exception( "Second number cannot be equal to zero.");
6. }
7. return (fNumber1 / fNumber2);
8. }
When implementing the Divide function we need to ensure that the user would not be able to set the "fNumber2" parameter (line 1) to zero as a division between zero does not exist. The validation statement in lines 3-6 takes care of this case but the important fact we need to notice is that this class will NEVER use specific methods to present errors like message boxes or writing into labels. Errors captured in the model part of the architecture ALWAYS have to be presented in the form of exceptions (line 5). This will allow us to use this object in several types of applications like ASP.NET applications, Windows applications, Web services, etc.
Once we have finished coding our Calculator class the project has to be built in order to get the .dll file we will use in our Web application.
MVC View-Controller Implementation
The View and the Controller objects will be implemented by using a common ASP.NET Website. Once we have created our project we need to add the reference to the .dll file we created before.
The option to do this can be found in the context menu when right-clicking the project in the solution explorer:
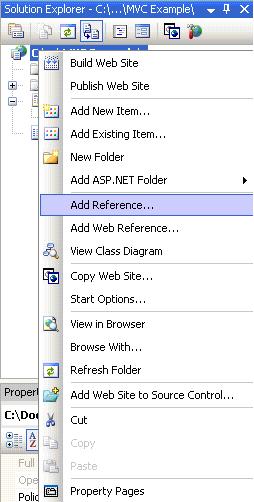
We can find the file in the path "\bin\Release" (or "\bin\Debug" depending on how you build your class library) inside our main folder containing the math class library project:
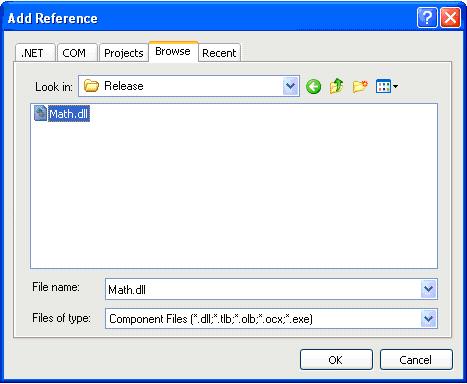
Once we have referenced our library we will create a simple web page that will allow us to choose between the four basic arithmetic operations and type two different numbers to operate.
The web page will look like this:
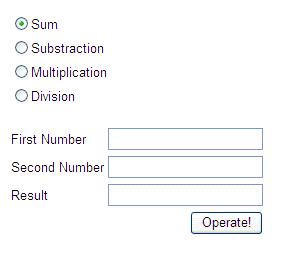
In the code behind we need to reference the Math namespace in order to use our Calculator class. The following statement will do that:
using Math;
As the code for this application is also simple we will only explain the method called when the "Operate!" button is clicked:
1. protected void btnOperate_Click(object sender, EventArgs e)
2. {
3. if (pbValidateNumbers())
4. {
5. Calculator cOperator = new Calculator();
6. try
7. {
8. txtResult.Text = cOperator.Operate(float.Parse(txtNumber1.Text.Trim()),float.Parse(txtNumber2.Text.Trim()), Convert.ToInt16(rblOperations.SelectedValue)).ToString();
9. lbError.Text = "";
10. }
11. catch (Exception ex)
12. {
13. txtResult.Text = "";
14. lbError.Text = ex.Message;
15. }
16. }
17.}
In line 3 we call the bool function "pbValidateNumbers" that will return true if the numbers typed in both textboxes are valid. These types of validations have to be performed by the controller object as they allow the interface to work properly and have nothing to do with the business logic.
In line 5 we create an instance of our Calculator class so we can perform the arithmetic operation. We call the method "Operate" (line 8) and return the value in another textbox. An important thing to mention is that we have to use a try-catch statement (lines 6-15) to handle any exception that could be thrown by our method "Operate" as every error caught in our Calculator class is handled by throwing a "digested" exception that is readable to the user.
In the code above we can appreciate how well encapsulated the business logic is, hence it can be reused in several applications without having to code it again.
Advantages of using MVC in ASP.NET
There's no duplicated code.
The business logic is encapsulated; hence the controller code is transparent and safer.
The business logic can be used in several front ends like Web pages, Web services, Windows applications, services, etc.
Exception handling is well managed showing the user only digested error messages.
Testing every part of an application is easier as it can be done separately using automated methods.
Application changes are easier to apply as they are focused in one part of the architecture only.